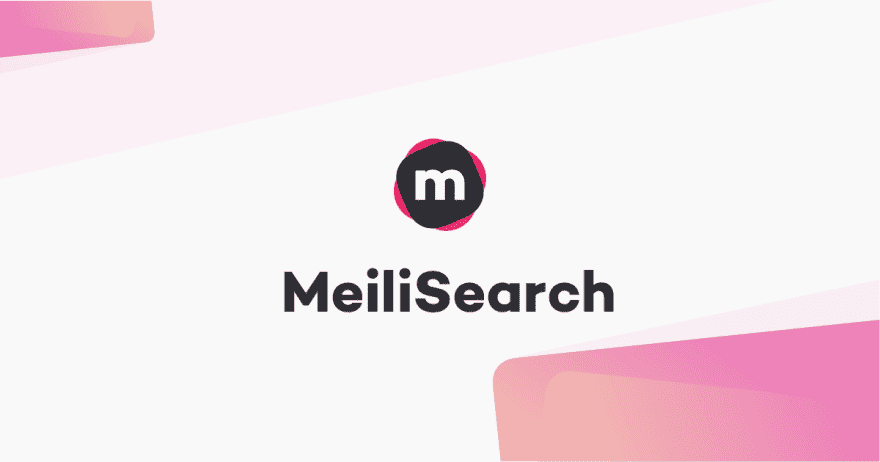
〔MeiliSearch〕Java 代码封装
大约 2 分钟
在 java 开发环境中,封装 meilisearch 工具。
代码
package com.lojob.meilisearch.service;
import com.alibaba.fastjson.JSON;
import com.lojob.domain.WebBook;
import com.meilisearch.sdk.Client;
import com.meilisearch.sdk.Index;
import com.meilisearch.sdk.SearchRequest;
import com.meilisearch.sdk.exceptions.MeilisearchException;
import com.meilisearch.sdk.model.*;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.List;
/**
* MeiliSearch工具类
*
* WebBook是meilisearch内存储的数据结构
*
* @author 小机灵鬼阅读
*/
@Service
public class MeiliSearchService implements InitializingBean, MeiliSearchOperations<WebBook> {
// 可排序属性
public static final String[] MEILISEARCH_SORTABLE = {"title", "appraise"};
private Index index;
@Resource
private Client client;
@Value("${meilisearch.uid}")
private String uid;
@Override
public Stats getStats() throws MeilisearchException {
return client.getStats();
}
@Override
public void clearIndex(String uid) throws MeilisearchException {
client.index(uid).deleteAllDocuments();
}
@Override
public WebBook get(String id) throws MeilisearchException {
return index.getDocument(id, WebBook.class);
}
@Override
public Results<WebBook> list() throws MeilisearchException {
DocumentsQuery query = new DocumentsQuery();
return index.getDocuments(query, WebBook.class);
}
@Override
public Results<WebBook> list(int offset, int limit) throws MeilisearchException {
DocumentsQuery query = new DocumentsQuery().setLimit(limit).setOffset(offset);
return index.getDocuments(query, WebBook.class);
}
@Override
public void add(List<WebBook> documents) throws MeilisearchException {
index.addDocuments(JSON.toJSONString(documents));
}
@Override
public void delete(String identifier) throws MeilisearchException {
index.deleteDocument(identifier);
}
/**
* 仅在标题下查询
*
* @param title 标题关键字
*/
@Override
public SearchResult search(String title) {
SearchRequest searchRequest = SearchRequest.builder().q(title).attributesToSearchOn(new String[]{"title"}).build();
return search(searchRequest);
}
/**
* 在标题和简介中查询,并按照appraise排序
*
* @param q 关键字
* @param classify 书籍类型
* @param offset 要跳过的文档数
* @param limit 返回的最大文档数
*/
@Override
public SearchResult search(String q, String classify, int offset, int limit) {
SearchRequest searchRequest = SearchRequest.builder().q(q).attributesToSearchOn(new String[]{"title", "synopsis"})
.attributesToHighlight(new String[]{"title", "synopsis"})
.highlightPreTag("<span class=\"highlight\">")
.highlightPostTag("</span>")
.sort(new String[]{"appraise:desc"})
.offset(offset)
.limit(limit)
.build();
if (!"all".equalsIgnoreCase(classify)) {
searchRequest.setFilterArray(new String[][]{
new String[]{"classify = " + classify}
});
}
return search(searchRequest);
}
/**
* 封装底层搜索
*
* @param sr 搜索条件
*/
@Override
public SearchResult search(SearchRequest sr) {
try {
return (SearchResult) index.search(sr);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
/**
* 初始化索引信息
*/
@Override
public void afterPropertiesSet() throws Exception {
index = client.index(uid);
Settings settings = new Settings();
// 设置可排序属性
settings.setSortableAttributes(MEILISEARCH_SORTABLE);
/**
* "words" 通过减少匹配的查询词数对结果进行排序
* "typo" 通过增加拼写错误的数量对结果进行排序
* "proximity" 通过增加匹配查询词之间的距离对结果进行排序
* "attribute" 根据属性排名顺序对结果进行排序
* "sort" 根据查询时确定的参数对结果进行排序
* "exactness" 根据匹配词与查询词的相似性对结果进行排序
*/
settings.setRankingRules(new String[]
{
"words",
"typo",
"proximity",
"attribute",
"sort",
"exactness"
});
settings.setFilterableAttributes(new String[]{"classify"});
index.updateSettings(settings);
System.out.println("初始化完成MeiliSearch的索引信息");
}
}